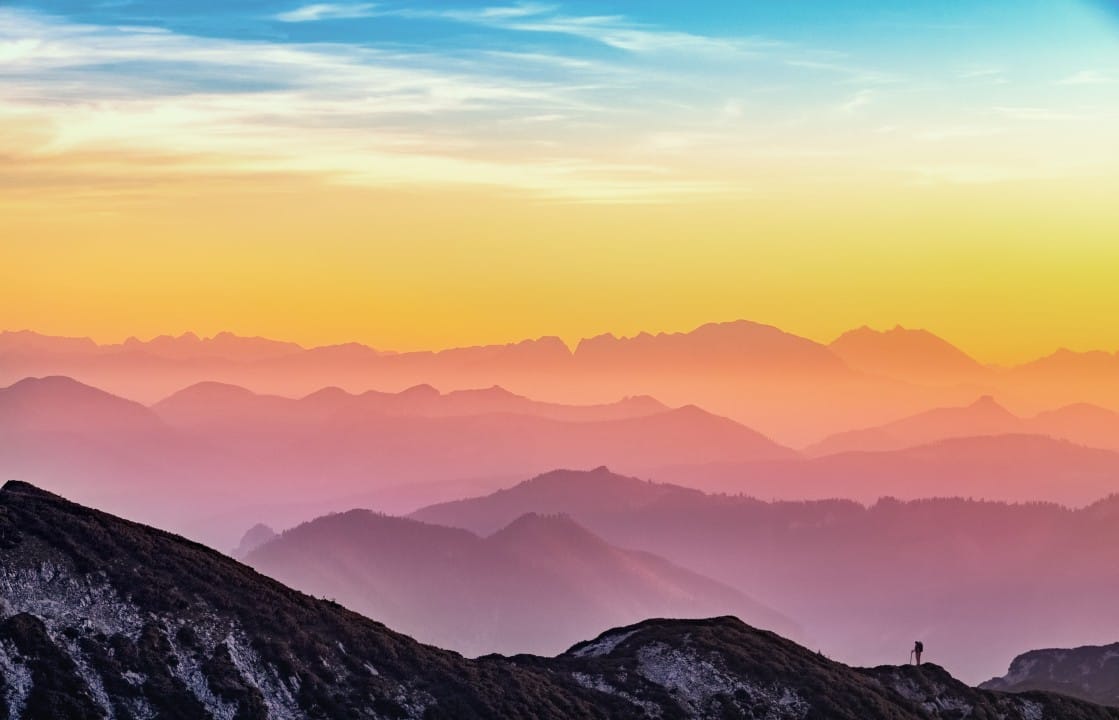
Parallax background animation in Reactjs + GSAP
If you are always wondering how other people create those eye catching website, the answer is simple. “They use animations in the right way.”
So, in this article, we not only show you how to create a parallax background on mouse movements, but we also show you how to think like a skilled Frontend developer, ultimately use animations and numbers together to make a stunning view!
creating a react app:
As always we need a react application, typescript and tailwind CSS for our work to be done. You can create it by yourself all from the start or just simply clone the bellow Github repository (master branch) which is a starter branch and consists of all the images we need as well.
GitHub - NekfarBaqir/parallax-bg-react
Before everything here is a demo of the app:
https://react-parallax-bg.vercel.app
When the project gets cloned, navigate inside it and open it in your favorite code editor. we will be using VS Code. then, install all the necessary dependencies by yarn, npm or pnpm. ( inside the project run the one of the bellow commands).
yarn
npm i
pnpm install
After installation, run the app and let’s get our hands dirty with developing animations:
Understanding what animation we are going to build:
The very first thing we need to do is to install a Library called GSAP (Green Sock animation Platform). This Library is has efficient JavaScript codes to create awesome animations ;moreover, it has a huge variety of plugins which make super complex animations easy for us.
yarn add gsap
When the package is installed now its time to understand the animation and how we are going to implement it. The animations is called parallax on mouse move. Basically, we are moving our mouse on the screen the background image is moving also; it could be the same direction as our mouse or not. So now let’s talk technically as a great Frontend Developer how to do it.
How?
For this scenario we should have a container context as in our example it is going to the div with ref of containerRef — this container will be useful for calculating the width and height of the aria we are moving our background image. The next thing we need is another div with an absolute position having the background image covered all the page (bgRef). Finally we listen to the mouse move and trigger an animation which change the background image position accordingly. Till this point lets see all these how in code:
.bash
import { useRef, useEffect } from "react";
import { parallaxIt } from "./animations";
import bg from "./assets/bg.jpg";
export default function App() {
const containerRef = useRef<HTMLDivElement>(null);
const bgRef = useRef<HTMLDivElement>(null);
let mouseMoveTimeOut: any;
useEffect(() => {
const bg = bgRef?.current;
const container = containerRef?.current;
if (container && bg) {
container.addEventListener("mousemove", (e) => {
if (mouseMoveTimeOut) clearTimeout(mouseMoveTimeOut);
setTimeout(() => {
parallaxIt(e, container, bg, -30);
}, 200);
});
}
}, [containerRef, bgRef]);
return (
<div
ref={containerRef}
className="h-screen w-screen overflow-hidden relative"
>
<div
ref={bgRef}
style={{ backgroundImage: `url(${bg})` }}
className="absolute inset-0 bg-blue-950 bg-cover bg-no-repeat bg-[20%] xl:bg-center bg-blend-overlay scale-105"
/>
</div>
);
}
Now in your browser you will get the error that parallaxIt can’t be imported as you guys didn’t import it yet.
okay!! let’s create the parallaxIt function and talk how it works.
I created a folder called animations inside src folder and created index.ts file. In that file I created parallaxIt function which takes e — mouse event object , container — reference to the container div, bg — reference to the background image div & a number value called movement — determine how far an item should repositioned as mouse moves. let’s take a look at its code an I will explain it more.
.bash
import { gsap, Power2 } from "gsap";
export const parallaxIt = (
e: MouseEvent,
containerRef: HTMLDivElement,
targetRef: HTMLDivElement,
movement: number
) => {
const locationX = e.pageX;
const LocationY = e.pageY;
const x =
((locationX - containerRef.offsetWidth / 2) / containerRef?.offsetWidth) *
movement;
const y =
((LocationY - containerRef.offsetHeight / 2) / containerRef.offsetHeight) *
movement;
gsap.to(targetRef, {
x: x,
y: y,
ease: Power2.easeOut,
});
};
As breif I can say, we simply track the mouse move and get it location, also; I get the context width and height. Finally I calculate a number for our background image to reposition. The above x and y variables will be calculated based on mouse location, container width/height and the movement ( minus mark changes the direction of the movement).
Alright guys now if you take a look at your browser, you can see a beautiful page and hovering it will create an stunning parallax animation.
if you wanted to see the full code here is the final version on the same repository ( complete branch).
🙏 Thanks for reading! If you liked it, stay tunned with JS Dojo news letter on Linkedin and share it with in your own community in daily.dev.
Also, If you want to be updated with the tools, insights and my everyday learning join my daily.dev squad & share your daily readings with all other JS developers: JS Dojo!